The Versatile init() Function in Go
The init() function in Go is a powerful tool for initializing package-level variables, registering packages, and performing one-time computations before the main program execution. It's important to use it judiciously, following best practices to maintain code readability and maintainability.
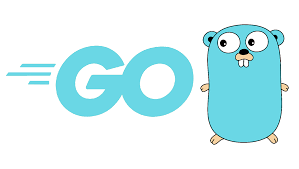
Introduction
Go, the modern and efficient programming language developed by Google, is known for its simplicity, concurrency, and powerful tooling. One of the unique features of Go is the init()
function, which plays a crucial role in the initialization and setup of Go programs. In this comprehensive article, we will delve into the intricacies of the init()
function, explore its various use cases, and discuss best practices for leveraging this powerful tool in your Go projects.
Understanding the init() Function
In Go, the init()
function is a special, predefined function that is automatically executed before the main()
function, which is the entry point of a Go program. The init()
function serves as a way to initialize the state of a package, perform one-time computations, or register a package with other parts of the application.
The init()
function has the following characteristics:
- Automatic Execution: The
init()
function is automatically called by the Go runtime before themain()
function is executed, ensuring that the necessary setup and initialization tasks are completed before the program starts running. - No Arguments or Return Values: The
init()
function does not take any arguments and does not return any values. It is designed to perform its tasks without any external input or output. - Multiple Occurrences: A Go package can have multiple
init()
functions, and they will be executed in the order they are declared within the package. This allows for modular and organized initialization logic. - Implicit Invocation: The
init()
function is never explicitly called by the developer. It is automatically invoked by the Go runtime, ensuring that the necessary setup is performed before the program starts.
Use Cases for the init() Function
The init()
function in Go can be utilized in a variety of scenarios, each with its own benefits and considerations. Let's explore some common use cases:
1. Initializing Package-Level Variables
One of the primary use cases for the init()
function is to initialize package-level variables that cannot be easily set during the declaration. This is particularly useful when the initialization process involves complex computations, reading from external sources, or relying on other package-level variables that need to be set first.
package main
import "fmt"
var greeting string
func init() {
greeting = "Hello, Go!"
}
func main() {
fmt.Println(greeting)
}
In this example, the greeting
variable is initialized in the init()
function, ensuring that it is ready for use in the main()
function.
2. Registering Packages and Side Effects
The init()
function can be used to register a package with other parts of the application, a technique known as "side effects." This is often used when a package needs to set up some global state or register itself with a central registry, ensuring that the program is aware of the package and can utilize its functionality correctly.
package main
import (
"fmt"
_ "example.com/mypackage"
)
func main() {
fmt.Println("Main function executed")
}
In this example, the mypackage
package is imported with the blank identifier (_
), which means that the package is imported solely for its side effects, i.e., the execution of its init()
function.
3. Performing One-Time Computations
The init()
function can be used to perform one-time computations or setup tasks that need to be executed before the program starts running. This is particularly useful when the initialization process is complex or time-consuming, and you want to ensure that it is completed before the main logic of the program begins.
package main
import (
"fmt"
"math/rand"
"time"
)
func init() {
rand.Seed(time.Now().UnixNano())
}
func main() {
fmt.Println("Random number:", rand.Intn(100))
}
In this example, the init()
function is used to seed the random number generator with the current time, ensuring that the random numbers generated in the main()
function are truly random.
4. Conditional Initialization
The init()
function can also be used to perform conditional initialization based on the runtime environment or other factors. This allows you to tailor the initialization process to specific scenarios, ensuring that the program is set up correctly for the given conditions.
package main
import (
"fmt"
"runtime"
)
func init() {
if runtime.GOOS == "linux" {
fmt.Println("This program will run on Linux.")
} else {
fmt.Println("This program will not run on Linux.")
}
}
func main() {
fmt.Println("Main function executed")
}
In this example, the init()
function checks the operating system and prints a message accordingly, allowing the program to adapt its behavior based on the runtime environment.
Best Practices for Using init()
While the init()
function is a powerful tool, it's important to use it judiciously and follow best practices to maintain the readability and maintainability of your Go code. Here are some guidelines to keep in mind:
- Minimize Side Effects: Avoid using the
init()
function to perform complex or time-consuming operations, as this can make the code harder to understand and debug. Limit theinit()
function to simple, straightforward initialization tasks. - Maintain Readability: Keep your
init()
functions concise and focused. If the initialization logic becomes too complex, consider breaking it down into smaller, more manageable functions or moving it to a separate package. - Avoid Relying on Order: While the order of
init()
function execution is determined by the order in which they are declared, it's generally better to avoid relying on this behavior. Instead, design your code to be self-contained and independent of the order in whichinit()
functions are executed. - Document Carefully: Provide clear and comprehensive documentation for your
init()
functions, explaining their purpose, the tasks they perform, and any dependencies or side effects they may have. This will help other developers understand and maintain your code. - Consider Alternative Approaches: In some cases, the
init()
function may not be the best solution, and alternative approaches, such as using aNew()
function or a dedicated initialization package, may be more appropriate. Evaluate the trade-offs and choose the approach that best fits your project's needs.
Conclusion
The init()
function in Go is a powerful and versatile tool that can greatly simplify the initialization and setup of your Go programs. By understanding its use cases, best practices, and potential pitfalls, you can leverage the init()
function to create more robust, maintainable, and efficient Go applications.
Remember, the init()
function is a powerful tool, but like any powerful tool, it should be used judiciously and with care. By following the guidelines and best practices outlined in this article, you can harness the full potential of the init()
function and write idiomatic, high-quality Go code.
Citations:
[1] https://www.digitalocean.com/community/tutorials/understanding-init-in-go
[2] https://www.developer.com/languages/inti-function-golang/
[3] https://go.dev/doc/effective_go
[4] https://tutorialedge.net/golang/the-go-init-function/
[5] https://www.geeksforgeeks.org/main-and-init-function-in-golang/