SmallestCSVParser: A Lightweight and Efficient CSV Parsing Library
The SmallestCSVParser is a lightweight and easy-to-use CSV parsing library for C# developers. It provides a simple API to read and parse CSV files according to the RFC4180 standard, without the complexity of other CSV parsing libraries.
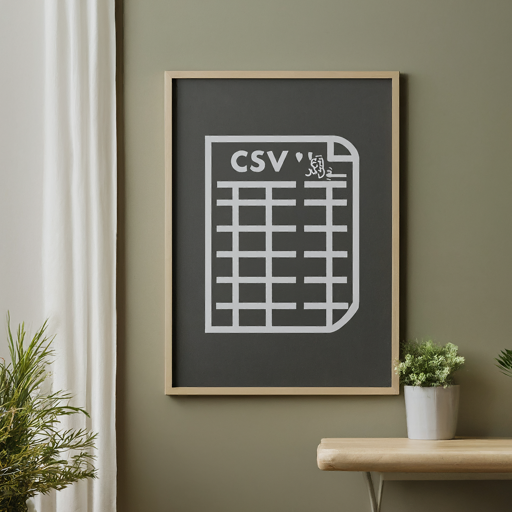
How to Use the Smallest CSV Parser in C#
The SmallestCSVParser is a lightweight and easy-to-use CSV parsing library for C# developers. It provides a simple API to read and parse CSV files according to the RFC4180 standard, without the complexity of other CSV parsing libraries.
Getting Started
To use the SmallestCSVParser, you first need to install the NuGet package:
dotnet add package SmallestCSVParser
Once the package is installed, you can start using the parser in your C# code:
using SmallestCSVParser;
var parser = new CSVParser();
while (true)
{
List<string>? columns = parser.ReadNextRow();
if (columns == null)
{
Console.WriteLine("End of file reached");
break;
}
var prettyRow = System.Text.Json.JsonSerializer.Serialize(columns);
Console.WriteLine($"Read row: {prettyRow}");
}
This code demonstrates the basic usage of the SmallestCSVParser. The ReadNextRow()
method returns a list of strings representing the columns in the current row of the CSV file. If the method returns null
, it means the end of the file has been reached.
Handling CSV Quirks
The SmallestCSVParser is designed to handle the common quirks and edge cases of CSV files, such as:
- Quoted fields: The parser correctly handles fields that contain commas or newlines by enclosing them in double quotes.
- Empty fields: The parser correctly handles empty fields, representing them as empty strings in the returned list.
- Trailing commas: The parser correctly handles CSV files with trailing commas at the end of rows.
Creating Custom Readers
The SmallestCSVParser provides a simple API, but you can easily build your own custom readers on top of it. For example, you can create a DictReader
that maps the column names to the corresponding values in each row:
using System.Collections.Generic;
using SmallestCSVParser;
public class DictReader
{
private readonly CSVParser _parser;
private List<string>? _headers;
public DictReader(string csvData)
{
_parser = new CSVParser(csvData);
_headers = _parser.ReadNextRow();
}
public Dictionary<string, string>? ReadNext()
{
var row = _parser.ReadNextRow();
if (row == null || _headers == null)
return null;
var dict = new Dictionary<string, string>();
for (int i = 0; i < row.Count; i++)
{
dict[_headers[i]] = row[i];
}
return dict;
}
}
This DictReader
class uses the SmallestCSVParser to read the CSV data and maps the column names to the corresponding values in each row, returning a Dictionary<string, string>
for each row.
Conclusion
The SmallestCSVParser is a lightweight and easy-to-use CSV parsing library for C# developers. It provides a simple API that adheres to the RFC4180 standard, making it a great choice for most CSV parsing needs. With its ability to handle common CSV quirks and the flexibility to build custom readers, the SmallestCSVParser is a valuable tool in any C# developer's toolkit.[1]
Citations:
[1] https://github.com/kjpgit/SmallestCSVParser