Mastering Multiline Strings in Golang: A Comprehensive Guide
Mastering Golang's multiline strings. Explore techniques, best practices, and practical applications for this powerful language feature.
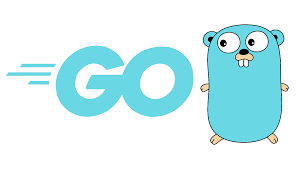
Introduction
As a programming language, Golang (also known as Go) has gained immense popularity in recent years, particularly in the realm of server-side development, cloud computing, and system programming. One of the many features that make Golang a powerful and versatile language is its handling of multiline strings. In this comprehensive guide, we'll explore the various techniques and best practices for working with multiline strings in Golang.
Understanding Multiline Strings in Golang
In Golang, multiline strings are represented using the backtick (`) character, also known as the "raw string literal" syntax. This approach allows you to create strings that span multiple lines without the need for concatenation or special escape characters.
Here's an example of a multiline string in Golang:
multilineString := `This is a
multiline
string.`
The resulting multilineString
variable will contain the following text:
This is a
multiline
string.
Multiline strings in Golang preserve the formatting and whitespace exactly as they appear in the code, making them a powerful tool for creating complex text-based content, such as configuration files, documentation, or even entire blocks of code.
Advantages of Multiline Strings in Golang
Using multiline strings in Golang offers several advantages over traditional string concatenation or the use of escape characters:
- Readability: Multiline strings make your code more readable and easier to maintain, as the structure of the text is clearly visible in the source code.
- Flexibility: Multiline strings allow you to easily incorporate complex formatting, such as indentation, line breaks, and even special characters, without the need for additional string manipulation.
- Efficiency: Compared to concatenating multiple single-line strings, multiline strings are more efficient in terms of memory usage and processing time, as they are stored and handled as a single entity.
- Consistency: By using multiline strings, you can ensure a consistent formatting and presentation of your text-based content across your Golang applications.
Practical Applications of Multiline Strings in Golang
Multiline strings in Golang have a wide range of practical applications, including:
1. Configuration Files
Multiline strings are particularly useful for storing and managing configuration files, such as YAML or JSON files, within your Golang applications. By using multiline strings, you can easily embed complex configuration data directly in your code, making it more maintainable and easier to distribute.
2. Documentation and Tutorials
Multiline strings are an excellent choice for creating rich, well-formatted documentation and tutorials within your Golang projects. You can use them to embed code samples, formatted text, and even images, providing a seamless and visually appealing experience for your users.
3. Template Generation
Multiline strings can be used to generate dynamic templates, such as HTML or email templates, within your Golang applications. This approach allows you to maintain the structure and formatting of your templates directly in your code, making them easier to manage and update.
4. Logging and Error Handling
When dealing with complex error messages or log entries, multiline strings can be a valuable tool for providing detailed and well-formatted information to your users or developers, improving the overall user experience and debugging process.
Techniques for Working with Multiline Strings in Golang
Now that we've covered the basics of multiline strings in Golang, let's dive into some practical techniques for working with them:
1. Embedding Code Samples
One of the most common use cases for multiline strings in Golang is the inclusion of code samples within your content. By using multiline strings, you can easily embed code snippets that maintain their formatting and syntax highlighting, making them more readable and engaging for your audience.
Here's an example of how you might use a multiline string to embed a Golang code sample:
codeExample := `
package main
import "fmt"
func main() {
multilineString := "This is a\nmultiline\nstring."
fmt.Println(multilineString)
}
`
2. Formatting with Indentation and Spacing
Multiline strings in Golang allow you to preserve the indentation and spacing of your text, making it easier to create well-formatted content. This can be particularly useful when working with structured data, such as configuration files or documentation.
formattedText := `
This is a multiline string
with consistent indentation.
The second line is indented
even further.
`
3. Incorporating Dynamic Content
Multiline strings in Golang can also be used to incorporate dynamic content, such as variables or function calls, into your text-based content. This can be achieved by using string interpolation, which allows you to embed Go expressions directly within your multiline strings.
name := "John Doe"
dynamicContent := `
Hello, my name is {{.name}}.
It's nice to meet you!
`
In this example, the {{.name}}
placeholder will be replaced with the value of the name
variable when the multiline string is rendered.
4. Handling Special Characters
Multiline strings in Golang provide a convenient way to handle special characters, such as quotes or backslashes, without the need for additional escaping. This can be particularly useful when working with text-based content that includes a lot of special characters, such as code samples or configuration files.
specialCharacters := `
This string includes "quotes" and a backslash: \
It's easy to include these characters in a multiline string.
`