Mastering Mockery: A Comprehensive Golang Tutorial
Master Mockery, a powerful Golang mocking framework, to write robust, testable, and maintainable code.
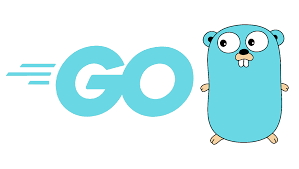
Introduction
Welcome to this comprehensive tutorial on Mockery, a powerful mocking framework for the Go programming language. Mockery is an essential tool in the arsenal of any Golang developer, as it enables you to write more robust, testable, and maintainable code.
In this tutorial, we will dive deep into the world of Mockery, exploring its features, use cases, and best practices. Whether you're a seasoned Golang developer or just starting your journey, this guide will equip you with the knowledge and skills to effectively leverage Mockery in your projects.
Understanding Mocking and Its Importance
Mocking is a software development technique that involves creating simulated or "mock" versions of external dependencies, such as APIs, databases, or third-party services. By using mocks, you can isolate the component you're testing and ensure that its behavior is independent of the behavior of its dependencies.
Mocking is particularly important in Golang development for several reasons:
- Testability: Mocks allow you to write more comprehensive and reliable tests, as you can simulate various scenarios and edge cases without relying on external systems.
- Faster Feedback Loops: By using mocks, you can run your tests quickly and efficiently, without waiting for external dependencies to respond or dealing with network latency.
- Maintainability: Mocks help you decouple your code, making it easier to refactor and update individual components without affecting the entire system.
- Reproducibility: Mocks ensure that your tests produce consistent and predictable results, regardless of the state of external dependencies.
Introducing Mockery
Mockery is a popular mocking framework for Golang that simplifies the process of creating and using mocks. It provides a simple and intuitive API for generating mock implementations of your interfaces, allowing you to focus on writing your tests rather than boilerplate code.
One of the key features of Mockery is its ability to automatically generate mock implementations based on your interface definitions. This means that you don't have to manually create and maintain mock implementations, which can be time-consuming and error-prone.
Installing and Setting Up Mockery
To get started with Mockery, you'll need to install the necessary tools. Mockery is available as a command-line tool, which you can install using the following command:
go get github.com/vektra/mockery/.../mockery
Once you have the mockery
command installed, you can use it to generate mock implementations for your interfaces.
Generating Mock Implementations
Suppose you have the following interface definition in your Golang project:
type UserService interface {
GetUser(id int) (*User, error)
CreateUser(user *User) error
UpdateUser(user *User) error
DeleteUser(id int) error
}
To generate a mock implementation for this interface, you can use the mockery
command:
mockery --name=UserService
This command will generate a new file, user_service_mock.go
, containing a mock implementation of the UserService
interface. The generated mock includes methods for each of the interface's methods, allowing you to customize the behavior of the mock as needed.
Using Mockery in Your Tests
Now that you have a mock implementation of the UserService
interface, you can use it in your tests to simulate various scenarios and test your code in isolation.
Here's an example of how you might use the generated mock in a test:
func TestUserService_GetUser(t *testing.T) {
// Create a new mock instance of the UserService
mockUserService := &mocks.UserService{}
// Set up the expected behavior of the mock
mockUserService.On("GetUser", 1).Return(&User{ID: 1, Name: "John Doe"}, nil)
// Call the method you're testing, passing in the mock instance
user, err := mockUserService.GetUser(1)
assert.NoError(t, err)
assert.Equal(t, &User{ID: 1, Name: "John Doe"}, user)
// Verify that the mock was called as expected
mockUserService.AssertExpectations(t)
}
In this example, we create a new mock instance of the UserService
interface, set up the expected behavior of the mock, and then use the mock in our test. We can also verify that the mock was called as expected using the AssertExpectations
method.
Advanced Mockery Features
Mockery offers a range of advanced features that can help you write more sophisticated and flexible tests. Here are a few examples:
Customizing Mock Behavior
In addition to setting up the expected behavior of a mock, you can also customize the mock's behavior to simulate different scenarios. For example, you can configure a mock to return an error, or to return different values based on the input parameters.
Partial Mocking
Mockery also supports partial mocking, which allows you to mock only a subset of an interface's methods. This can be useful when you want to test the behavior of a specific method in isolation, while still using the real implementation of the other methods.
Argument Matching
Mockery provides a range of argument matching options, which allow you to specify more complex expectations for the input parameters of your mock methods. This can be particularly useful when you're dealing with complex data structures or when you need to verify that your code is handling specific edge cases correctly.
Stubbing and Spying
In addition to mocking, Mockery also supports stubbing and spying. Stubbing allows you to override the behavior of a method, while spying allows you to observe the interactions between your code and its dependencies without modifying their behavior.
Best Practices for Using Mockery
To get the most out of Mockery and ensure that your tests are effective and maintainable, it's important to follow a few best practices:
- Keep Mocks Simple: Avoid creating overly complex mocks, as this can make your tests harder to understand and maintain.
- Separate Concerns: Try to keep your mocks focused on a specific set of dependencies, rather than trying to mock everything in a single test.
- Use Meaningful Names: When generating mock implementations, use meaningful names that clearly describe the purpose of the mock.
- Document Your Mocks: Provide clear documentation for your mocks, explaining their purpose, expected behavior, and any relevant edge cases.
- Avoid Overusing Mocks: While mocks are a powerful tool, it's important not to overuse them. Make sure to balance the use of mocks with integration tests that exercise your system as a whole.
- Keep Mocks Up-to-Date: Regularly review and update your mocks to ensure that they accurately reflect the behavior of your real dependencies.
Real-World Examples and Use Cases
To help you better understand how to use Mockery in your own projects, let's explore a few real-world examples and use cases.
Example 1: Testing a Web API
Suppose you're building a web API that interacts with a user service. You can use Mockery to create a mock implementation of the UserService
interface, allowing you to test your API endpoints in isolation without relying on the actual user service.
func TestUserController_GetUser(t *testing.T) {
// Create a new mock instance of the UserService
mockUserService := &mocks.UserService{}
// Set up the expected behavior of the mock
mockUserService.On("GetUser", 1).Return(&User{ID: 1, Name: "John Doe"}, nil)
// Create a new instance of the UserController, passing in the mock UserService
controller := NewUserController(mockUserService)
// Call the GetUser endpoint and assert the expected response
w := httptest.NewRecorder()
r := httptest.NewRequest("GET", "/users/1", nil)
controller.GetUser(w, r)
assert.Equal(t, http.StatusOK, w.Code)
assert.Equal(t, `{"id":1,"name":"John Doe"}`, w.Body.String())
// Verify that the mock was called as expected
mockUserService.AssertExpectations(t)
}
Example 2: Testing a Database-Backed Service
Suppose you have a service that interacts with a database to perform CRUD operations on user data. You can use Mockery to create a mock implementation of the database client, allowing you to test your service without actually connecting to the database.
func TestUserService_CreateUser(t *testing.T) {
// Create a new mock instance of the database client
mockDB := &mocks.DatabaseClient{}
// Set up the expected behavior of the mock
mockDB.On("Insert", "users", &User{Name: "John Doe"}).Return(1, nil)
// Create a new instance of the UserService, passing in the mock database client
service := NewUserService(mockDB)
// Call the CreateUser method and assert the expected result
user := &User{Name: "John Doe"}
err := service.CreateUser(user)
assert.NoError(t, err)
assert.Equal(t, 1, user.ID)
// Verify that the mock was called as expected
mockDB.AssertExpectations(t)
}
Example 3: Testing a Third-Party API Integration
Suppose your application integrates with a third-party API, such as a payment gateway or a weather service. You can use Mockery to create a mock implementation of the API client, allowing you to test your integration code without relying on the actual API.
func TestPaymentService_ProcessPayment(t *testing.T) {
// Create a new mock instance of the payment API client
mockPaymentAPI := &mocks.PaymentAPIClient{}
// Set up the expected behavior of the mock
mockPaymentAPI.On("ProcessPayment", &Payment{Amount: 100.00}).Return(&PaymentResponse{
ID: "123456789",
Status: "success",
}, nil)
// Create a new instance of the PaymentService, passing in the mock API client
service := NewPaymentService(mockPaymentAPI)
// Call the ProcessPayment method and assert the expected result
payment := &Payment{Amount: 100.00}
response, err := service.ProcessPayment(payment)
assert.NoError(t, err)
assert.Equal(t, "123456789", response.ID)
assert.Equal(t, "success", response.Status)
// Verify that the mock was called as expected
mockPaymentAPI.AssertExpectations(t)
}
These examples demonstrate how Mockery can be used to simplify the testing of various components in a Golang application, from web APIs to database-backed services and third-party API integrations.
Conclusion
In this comprehensive tutorial, we've explored the power of Mockery, a powerful mocking framework for Golang. We've covered the importance of mocking, the key features of Mockery, and how to use it effectively in your own projects.
By leveraging Mockery, you can write more robust, testable, and maintainable Golang code, ensuring that your applications are reliable and scalable. Whether you're a seasoned Golang developer or just starting your journey, this tutorial has provided you with the knowledge and tools you need to master the art of mocking with Mockery.
So, go forth and conquer your testing challenges with the help of Mockery!