Mastering JSON Handling in Golang: A Comprehensive Guide
Golang, with its emphasis on simplicity, efficiency, and strong typing, is an excellent choice for working with JSON data.
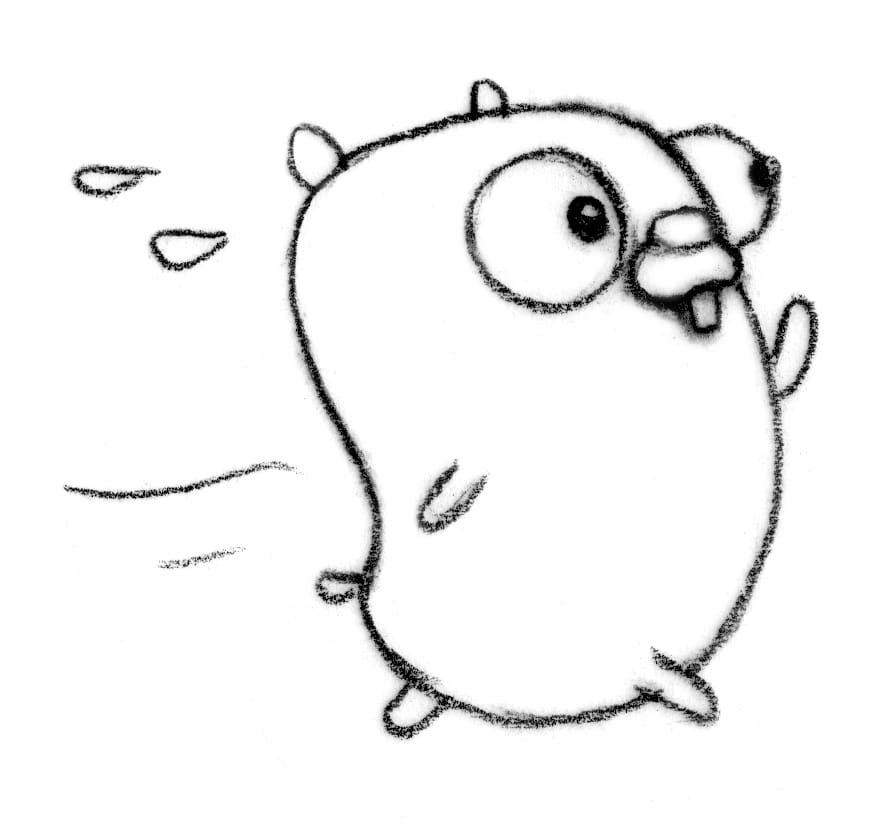
Golang, with its emphasis on simplicity, efficiency, and strong typing, is an excellent choice for working with JSON data. Whether you're building web applications, integrating with APIs, or storing data in databases, Golang's standard library provides a robust set of tools for encoding, decoding, marshaling, and unmarshaling JSON.
In this comprehensive guide, we'll explore the various aspects of JSON handling in Golang, from the fundamentals to advanced techniques, equipping you with the knowledge and best practices to effectively manage JSON data in your Golang projects.
The Basics of JSON Handling in Golang
Golang's standard library includes the encoding/json
package, which serves as the primary interface for working with JSON data. This package provides a straightforward and intuitive API for encoding Golang data structures to JSON and decoding JSON data into Golang data structures.
Encoding Golang Data to JSON
The json.Marshal()
function is the workhorse for encoding Golang data to JSON. This function takes an interface{} as input and returns a byte slice representing the JSON-encoded data, along with any errors that may have occurred during the encoding process.
type Person struct {
Name string
Age int
}
person := Person{
Name: "John Doe",
Age: 30,
}
jsonData, err := json.Marshal(person)
if err != nil {
// Handle the error
return
}
fmt.Println(string(jsonData)) // Output: {"Name":"John Doe","Age":30}
In this example, we define a Person
struct and create an instance of it. We then use the json.Marshal()
function to encode the person
object to a JSON-formatted byte slice, which we convert to a string for printing.
Decoding JSON Data to Golang
The counterpart to json.Marshal()
is json.Unmarshal()
, which allows you to decode JSON data into Golang data structures. This function takes a byte slice of JSON data and a pointer to a Golang data structure, which will be populated with the decoded data.
jsonData := []byte(`{"Name":"John Doe","Age":30}`)
var person Person
err := json.Unmarshal(jsonData, &person)
if err != nil {
// Handle the error
return
}
fmt.Println(person.Name) // Output: John Doe
fmt.Println(person.Age) // Output: 30
In this example, we define a JSON-formatted byte slice and use json.Unmarshal()
to decode it into a Person
struct. The decoded data is then accessible through the person
variable.
Customizing JSON Encoding and Decoding
While the default behavior of the encoding/json
package is often sufficient, Golang provides several ways to customize the JSON encoding and decoding process to suit your specific needs.
Struct Tags
Golang's struct tags allow you to control how fields in a struct are encoded and decoded. You can use the json
struct tag to specify the name of the field in the JSON output, as well as other options like omitting empty fields or specifying a default value.
type Person struct {
FullName string `json:"name"`
Age int `json:"age,omitempty"`
Email string `json:"-"`
}
person := Person{
FullName: "John Doe",
Age: 30,
Email: "john.doe@example.com",
}
jsonData, err := json.Marshal(person)
if err != nil {
// Handle the error
return
}
fmt.Println(string(jsonData)) // Output: {"name":"John Doe","age":30}
In this example, we use the json
struct tag to customize the field names in the JSON output, omit the Age
field if it's zero, and exclude the Email
field entirely.
Custom Marshalers and Unmarshalers
If the struct tag customizations are not enough, you can implement the json.Marshaler
and json.Unmarshaler
interfaces on your own types to provide custom encoding and decoding logic.
type Person struct {
Name string
Age int
}
func (p *Person) MarshalJSON() ([]byte, error) {
return []byte(fmt.Sprintf(`{"name":"%s","age":%d}`, p.Name, p.Age)), nil
}
func (p *Person) UnmarshalJSON(data []byte) error {
var v map[string]interface{}
if err := json.Unmarshal(data, &v); err != nil {
return err
}
p.Name, _ = v["name"].(string)
p.Age, _ = v["age"].(int)
return nil
}
person := &Person{
Name: "John Doe",
Age: 30,
}
jsonData, err := json.Marshal(person)
if err != nil {
// Handle the error
return
}
fmt.Println(string(jsonData)) // Output: {"name":"John Doe","age":30}
In this example, we implement the MarshalJSON()
and UnmarshalJSON()
methods on the Person
struct to provide custom JSON encoding and decoding logic. This allows us to control the exact format of the JSON output and handle any necessary data transformations during the decoding process.
Handling Complex JSON Structures
Real-world JSON data often involves more complex structures, such as nested objects, arrays, and heterogeneous data types. Golang's encoding/json
package provides seamless support for working with these complex JSON structures.
Nested Structures
Nested JSON structures can be easily mapped to Golang structs with nested fields.
type Address struct {
Street string
City string
Country string
}
type Person struct {
Name string
Age int
Address Address
}
person := Person{
Name: "John Doe",
Age: 30,
Address: Address{
Street: "123 Main St",
City: "Anytown",
Country: "USA",
},
}
jsonData, err := json.Marshal(person)
if err != nil {
// Handle the error
return
}
fmt.Println(string(jsonData)) // Output: {"Name":"John Doe","Age":30,"Address":{"Street":"123 Main St","City":"Anytown","Country":"USA"}}
In this example, we define a Person
struct with a nested Address
struct. When we marshal the person
object to JSON, the nested structure is automatically encoded.
JSON Arrays
Golang slices and arrays can be easily encoded and decoded to and from JSON arrays.
type Person struct {
Name string
Hobbies []string
}
person := Person{
Name: "John Doe",
Hobbies: []string{"reading", "hiking", "cooking"},
}
jsonData, err := json.Marshal(person)
if err != nil {
// Handle the error
return
}
fmt.Println(string(jsonData)) // Output: {"Name":"John Doe","Hobbies":["reading","hiking","cooking"]}
In this example, we define a Person
struct with a slice of strings for the Hobbies
field. When we marshal the person
object to JSON, the Hobbies
slice is automatically encoded as a JSON array.
Heterogeneous Data
Golang's interface{}
type can be used to handle JSON data with heterogeneous data types, such as objects, arrays, and primitive values.
var jsonData = []byte(`{"name":"John Doe","age":30,"hobbies":["reading","hiking","cooking"],"address":{"street":"123 Main St","city":"Anytown","country":"USA"}}`)
var v interface{}
err := json.Unmarshal(jsonData, &v)
if err != nil {
// Handle the error
return
}
m := v.(map[string]interface{})
fmt.Println(m["name"]) // Output: John Doe
fmt.Println(m["age"]) // Output: 30.0 (note the type is float64)
fmt.Println(m["hobbies"]) // Output: [reading hiking cooking]
fmt.Println(m["address"]) // Output: map[city:Anytown country:USA street:123 Main St]
In this example, we use an interface{}
to unmarshal the JSON data into a generic map. We can then access the individual fields and their respective data types using type assertions.
Performance Considerations
While the standard encoding/json
package provides a solid foundation for JSON handling in Golang, there are situations where you may want to optimize the performance of your JSON processing. Golang has several third-party JSON libraries that offer enhanced performance, flexibility, and features.
High-Performance JSON Libraries
Some popular high-performance JSON libraries for Golang include:
- goccy/go-json: A drop-in replacement for
encoding/json
that offers significant performance improvements, especially for encoding and decoding large JSON payloads. - json-iterator/go: A high-performance JSON library that provides a compatible API with
encoding/json
, while offering additional features and optimizations. - easyjson: A fast JSON serializer for Golang that generates custom JSON marshalers to achieve high performance.
These libraries often use techniques like code generation, SIMD instructions, and other low-level optimizations to achieve significant performance gains over the standard encoding/json
package.
Benchmarking and Profiling
When working with large or complex JSON data, it's important to benchmark your JSON processing code to identify potential performance bottlenecks. Golang provides excellent tooling for profiling and optimizing your code, including the built-in pprof
package and third-party tools like go-critic and go-fuzz.
By leveraging these tools, you can identify and address performance issues in your JSON handling code, ensuring that your Golang applications can efficiently process even the most demanding JSON workloads.
Conclusion
Golang's encoding/json
package provides a robust and easy-to-use set of tools for working with JSON data. From the fundamentals of encoding and decoding JSON to advanced techniques for handling complex structures and optimizing performance, this guide has covered the essential aspects of JSON handling in Golang.
By mastering these concepts and best practices, you'll be well-equipped to manage JSON data in your Golang projects, leading to more efficient, maintainable, and error-free code. As you continue to explore and experiment with Golang's JSON capabilities, remember to stay up-to-date with the latest developments in the Golang ecosystem, as new libraries and tools may emerge to further enhance your JSON processing capabilities.
Citations:
[1] https://betterstack.com/community/guides/scaling-go/json-in-go/
[2] https://www.reddit.com/r/golang/comments/16o3myq/how_i_made_my_highperformance_json_library_even/?rdt=58026
[3] https://blog.logrocket.com/using-json-go-guide/
[4] https://go.dev/blog/json
[5] https://github.com/goccy/go-json