How to Integrate Elasticsearch into a Golang Server: A Comprehensive Guide
Integrate Elasticsearch into your Golang server for powerful search capabilities. Set up Elasticsearch, connect using the official client library, and index/search documents efficiently. Explore advanced topics like mapping strategies, scaling, security, performance tuning, and integration.
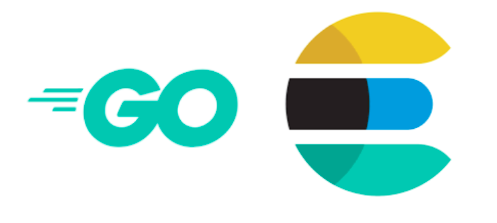
Introduction
Elasticsearch is a powerful open-source search and analytics engine that provides a distributed, scalable, and high-performance full-text search solution. Integrating Elasticsearch into your Golang server can significantly enhance the search capabilities of your application, enabling fast and accurate search results for your users. This comprehensive guide will walk you through the process of setting up Elasticsearch, indexing data, performing searches, and exploring advanced features for optimal performance and scalability.
Understanding Elasticsearch
Before diving into the integration process, it's crucial to understand the fundamental concepts of Elasticsearch. Elasticsearch organizes data into indices, which contain one or more types, similar to tables in a relational database. Documents, the basic units of data, are stored within these types. Elasticsearch provides a RESTful API for indexing data, searching for documents, and managing indices, supporting various query types and aggregations.
Setting up Elasticsearch
You can either install Elasticsearch locally or use a managed service like Elastic Cloud or Amazon Elasticsearch Service. If installing locally, download the appropriate package from the official Elasticsearch website and follow the installation instructions.
Integrating Elasticsearch with Golang
To integrate Elasticsearch with your Golang server, you'll need to use a client library like the official Elasticsearch client library provided by Elastic.
Installing the Client Library
go get github.com/elastic/go-elasticsearch
Connecting to Elasticsearch
cfg := elasticsearch.Config{
Addresses: []string{"http://localhost:9200"},
}
es, err := elasticsearch.NewClient(cfg)
if err != nil {
log.Fatalf("Error creating Elasticsearch client: %s", err)
}
Indexing Data
type Document struct {
Title string `json:"title"`
Content string `json:"content"`
}
func indexDocument(es *elasticsearch.Client, doc Document) error {
body, err := json.Marshal(doc)
if err != nil {
return err
}
req := esapi.IndexRequest{
Index: "my-index",
DocumentID: "1",
Body: bytes.NewReader(body),
}
res, err := req.Do(context.Background(), es)
if err != nil {
return err
}
defer res.Body.Close()
if res.IsError() {
log.Printf("Error indexing document: %s", res.String())
} else {
log.Println("Document indexed successfully")
}
return nil
}
Searching for Documents
func searchDocuments(es *elasticsearch.Client, query string) error {
var buf bytes.Buffer
query := map[string]interface{}{
"query": map[string]interface{}{
"multi_match": map[string]interface{}{
"query": query,
"fields": []string{"title", "content"},
"operator": "and",
},
},
}
if err := json.NewEncoder(&buf).Encode(query); err != nil {
return err
}
res, err := es.Search(
es.Search.WithContext(context.Background()),
es.Search.WithIndex("my-index"),
es.Search.WithBody(&buf),
es.Search.WithTrackTotalHits(true),
es.Search.WithPretty(),
)
if err != nil {
return err
}
defer res.Body.Close()
if res.IsError() {
var e map[string]interface{}
if err := json.NewDecoder(res.Body).Decode(&e); err != nil {
return err
}
log.Printf("Error searching for documents: %s", e)
} else {
var r map[string]interface{}
if err := json.NewDecoder(res.Body).Decode(&r); err != nil {
return err
}
fmt.Printf("Search results: %+v\n", r)
}
return nil
}
Advanced Topics
Indexing Strategies
Bulk Indexing
For indexing large amounts of data efficiently, use the Elasticsearch bulk API:
bulkRequest := esapi.BulkRequest{
Body: bytes.NewReader(bulkBody),
Refresh: "true",
}
res, err := bulkRequest.Do(context.Background(), es)
Indexing Pipelines
Elasticsearch indexing pipelines allow data transformations and enrichment during indexing for tasks like normalization and language analysis.
Index Aliases
Index aliases group multiple indices under a single name, enabling seamless index switching for reindexing or migrations.
Querying and Aggregations
Query Context
Set query contexts to control timeouts, termination conditions, and result filtering:
res, err := es.Search(
es.Search.WithContext(context.Background()),
es.Search.WithTrackTotalHits(true),
es.Search.WithTerminateAfter(10000),
)
Pagination
Implement pagination using the from
and size
parameters for large result sets.
Aggregations
Perform powerful data analysis and metrics calculation using Elasticsearch's aggregation capabilities, including bucket, metric, and pipeline aggregations.
Cluster Management
Cluster Health
Monitor cluster health using the cluster health API for status, node counts, and potential issues.
Snapshots and Restore
Create snapshots of indices for backups or restoring data to a new cluster, configuring snapshot repositories and lifecycle policies.
Cross-Cluster Replication
For geographically distributed deployments, use cross-cluster replication to replicate indices between clusters, ensuring data availability and reducing latency.
Security and Monitoring
Authentication and Access Control
Implement authentication mechanisms (e.g., basic auth, API keys) and role-based access control for managing user permissions.
Monitoring and Alerting
Monitor Elasticsearch metrics like cluster health, node statistics, and indexing performance using tools like Elasticsearch Watcher, Prometheus, and Grafana.
Audit Logging
Enable audit logging to record user actions and system events for security and compliance purposes.
Here are some additional details and advanced topics to further enhance the Elasticsearch integration with your Golang server:
Mapping and Indexing Strategies
Field Mappings
Elasticsearch allows you to define mappings for your indices, specifying the structure and data types of the fields in your documents. Proper mapping can significantly improve search performance and accuracy. You can define field mappings explicitly or let Elasticsearch automatically infer them based on the data you index.
mapping := `{
"properties": {
"title": {
"type": "text"
},
"content": {
"type": "text"
},
"published_date": {
"type": "date"
}
}
}`
req := esapi.IndicesCreateRequest{
Index: "my-index",
Body: strings.NewReader(mapping),
}
res, err := req.Do(context.Background(), es)
Parent-Child Relationships
Elasticsearch supports parent-child relationships, which can be useful when dealing with nested or hierarchical data structures. This feature allows you to index child documents as part of their parent document, enabling efficient queries and aggregations based on the parent-child relationship.
Nested Objects
For complex data structures with nested objects, Elasticsearch provides the nested data type. This allows you to index and query nested objects as separate entities while still maintaining their relationship with the root document.
Dynamic Mappings
Elasticsearch can automatically detect and map new fields in your documents, a feature known as dynamic mappings. While convenient, this can lead to unexpected mapping types and potential performance issues. It's recommended to explicitly define your mappings or disable dynamic mappings in production environments.
Scaling and Performance Tuning
Sharding and Replication
Elasticsearch supports horizontal scaling by distributing data across multiple shards, which are then replicated for high availability and fault tolerance. You can configure the number of shards and replicas for each index based on your data volume and performance requirements.
Indexing Buffer
Elasticsearch uses an indexing buffer to batch and optimize write operations. You can tune the indexing buffer settings, such as the size and flush intervals, to balance indexing performance and resource utilization.
Query Caching
Elasticsearch provides a query cache that can significantly improve the performance of frequently executed queries. You can enable and configure the query cache settings based on your application's query patterns and memory constraints.
Circuit Breakers
Elasticsearch uses circuit breakers to prevent out-of-memory errors and ensure system stability. You can adjust the circuit breaker settings to control the amount of memory allocated for various operations, such as fielddata and request processing.
Monitoring and Alerting
Elasticsearch Monitoring
Elasticsearch provides built-in monitoring capabilities through the Monitoring APIs, allowing you to track various metrics and statistics related to your cluster, nodes, indices, and queries. You can integrate these APIs with monitoring tools like Kibana or third-party solutions for comprehensive monitoring and alerting.
Logging and Auditing
In addition to monitoring, Elasticsearch supports extensive logging and auditing capabilities. You can configure various log levels and formats to capture detailed information about indexing, searching, and cluster operations. Audit logging can be enabled to track user actions and system events for security and compliance purposes.
Integration with Other Technologies
Logstash and Beats
Logstash and Beats are part of the Elastic Stack and can be used to collect, process, and ship data from various sources to Elasticsearch. Logstash provides a powerful data processing pipeline, while Beats are lightweight shippers for efficiently sending data to Elasticsearch or Logstash.
Kibana
Kibana is a powerful data visualization and exploration tool that integrates seamlessly with Elasticsearch. It provides a user-friendly interface for querying, analyzing, and visualizing data stored in Elasticsearch indices.
Elasticsearch Clients for Other Languages
While this guide focuses on integrating Elasticsearch with a Golang server, Elasticsearch provides official client libraries for various programming languages, including Java, Python, Ruby, and more. This allows you to integrate Elasticsearch into applications written in different languages or build polyglot systems.
By leveraging these advanced features, mapping and indexing strategies, scaling techniques, monitoring and alerting capabilities, and integrations with other technologies, you can build a robust and high-performance Elasticsearch integration within your Golang server. This will enable you to deliver a seamless and efficient search experience while ensuring scalability, reliability, and maintainability for your application.
Conclusion
Integrating Elasticsearch into your Golang server can significantly enhance your application's search capabilities, providing fast and accurate search results to your users. By following this comprehensive guide, you'll learn how to set up Elasticsearch, index data, perform searches, and leverage advanced features for optimal performance and scalability. With its powerful search and analytics capabilities, Elasticsearch can be a valuable addition to your Golang server, enabling you to deliver a seamless and efficient search experience.